운영체제 - 김종익교수님
프로세스
실행 중인 프로그램
- text section : 코드가 있는 부분
- Stack : temporary data를 담고 있다. 함수 파라미터, return주소, 지역변수
- Data section : 프로그램의 시작부터 끝까지 유지되는 전역변수
- 초기화 된 데이터
- 초기화 되지 않은 데이터
- Heap : 실행 중에 동적으로 메모리 할당(new, malloc)
Program Counter
다음 수행할 프로그램 코드의 위치를 가리키는 register value
현재 작업하고 있는 정보들은 CPU register에 다 들어있다.
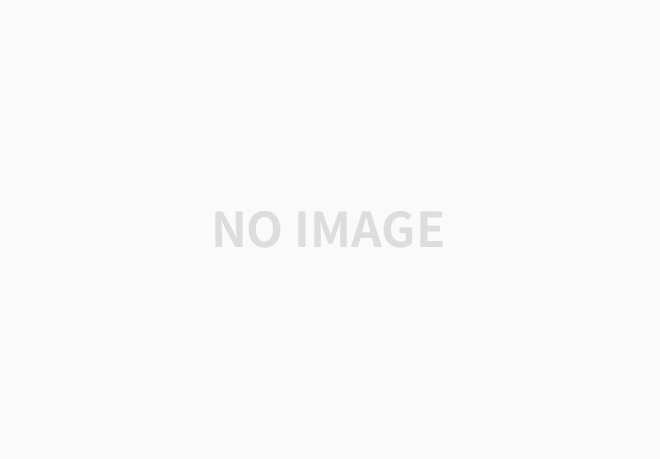
프로세스의 상태
1. new
2. running
3. waiting
4. ready
5. terminated
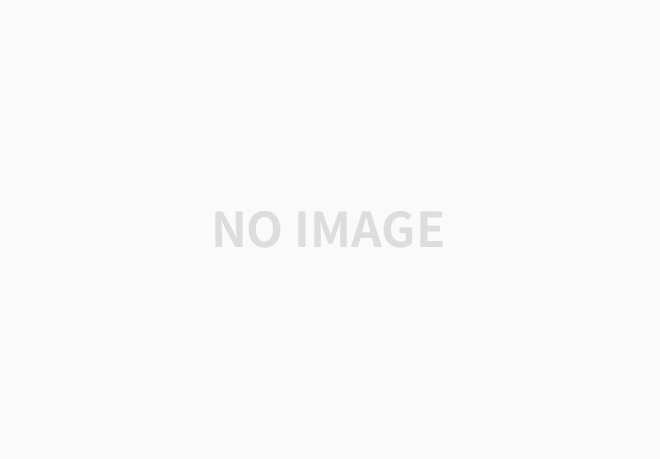
PCB(Process Control Block)
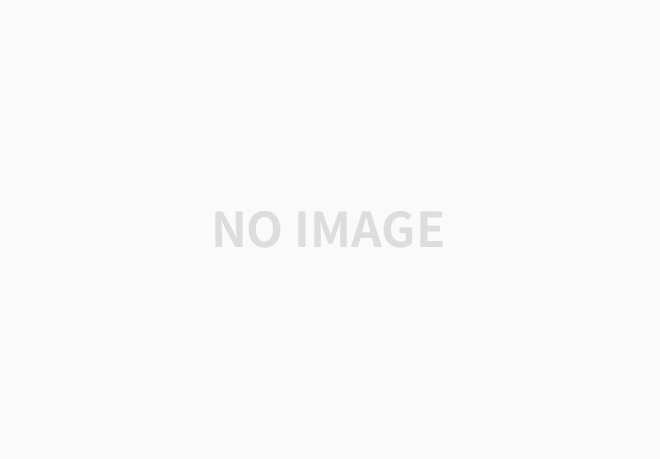
CPU Switch From Process to Process
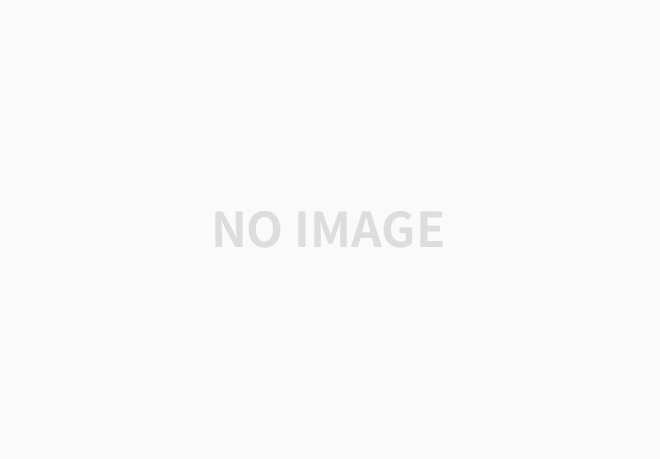
Job queue : CPU에 있는 모든 프로세스
Ready queue : ready상태에 있는 프로세스만
Device queues : 디바이스마다 존재
Process 생성
parent process가 children processes를 만듦
=> 트리 형태의 계층구조를 가지게 됨
프로세스는 pid를 가지고 있어 이 숫자로 구분된다.
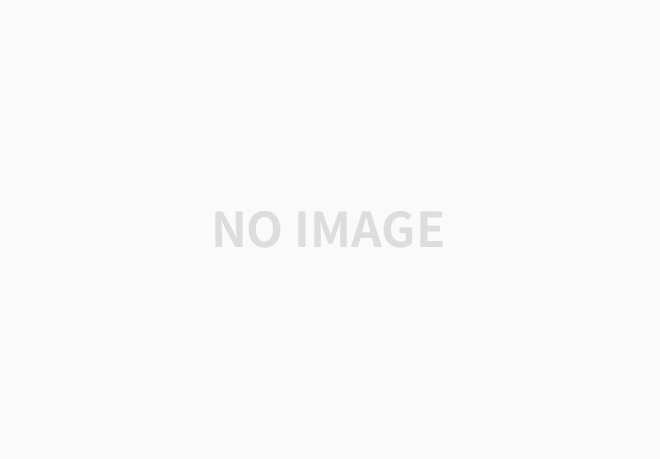
부모 프로세스가 wait하지 않는 경우 => 자식 프로세스는 zombie 상태
부모 프로세스가 wait을 하지 않고 먼저 종료하는 경우 => 자식 프로세스는 orphan 상태
zombie => 시스템 리소스를 잡고 실행하지 못하게 하도록 한다.
IPC(Interprocess Communication)
프로세스 간 사이에 정보를 주고 받을 수 있는
1. shared memory
2. message processing
Pipes
한쪽 방향으로만 데이터가 흘러갈 수 있다.
parent-child 관계에서만 만들어지는 파이프
Threads
- many to one
- one to one
- many to many
팀프로젝트 - 프로토타입 구현 회의
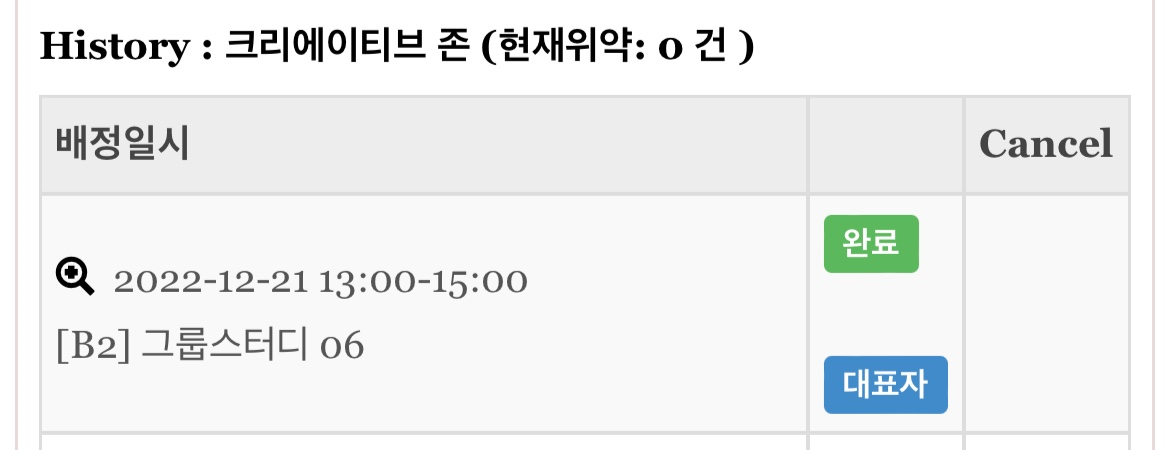
도서관 크리에이티브 존에서 13:00 - 15:00 회의 진행
프로토타입 구현 및 프로젝트의 상세 기능에 대해 회의를 진행하였다.
피그마의 프로토타입 기능을 활용하여 화면 이동까지 구현을 완료하였다.
코딩테스트
계산기 구현하기
import java.util.*;
import java.io.*;
class Solution {
static class Operand {
int priority;
char op;
Operand(int priority, char op) {
this.priority = priority;
this.op = op;
}
}
static Stack<Operand> op = new Stack<>();
static Stack<Integer> num = new Stack<>();
static boolean isOp(char c) {
switch(c) {
case '+':
case '-':
case '*':
case '/':
case '(':
case ')':
return true;
default:
return false;
}
}
static int getPriority(char c) {
switch(c) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
static boolean isPair(char left, char right) {
if (left == '(' && right == ')') {
return true;
}
return false;
}
static int calc(int num1, char op, int num2) {
if(op == '+') {
return num1 + num2;
}
else if (op == '-') {
return num1 - num2;
}
else if (op == '*') {
return num1 * num2;
}
else if (op == '/') {
return num1 / num2;
}
return -1;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String s = sc.nextLine();
char[] ss = s.toCharArray();
String n = "";
for(int i = 0 ; i < ss.length; i++) {
if(!isOp(ss[i])) {
//여러자리 숫자인 경우 처리 필요
n += Character.toString(ss[i]);
} else {
if(!n.equals("")) {
num.push(Integer.parseInt(n));
n = "";
}
//operator
if(ss[i] == '(') {
op.push(new Operand(0, ss[i]));
} else if(ss[i] == ')') {
Operand o = op.pop();
//구현
while(!op.isEmpty()) {
if(isPair(o.op, ss[i])) {
break;
}
int num2 = num.pop();
int num1 = num.pop();
int result = calc(num1, o.op, num2);
num.push(result);
o = op.pop();
}
} else {
int priority = getPriority(ss[i]);
if(op.isEmpty()) {
op.push(new Operand(priority, ss[i]));
} else {
while(!op.isEmpty()) {
if (op.peek().priority >= priority) {
int num2 = num.pop();
int num1 = num.pop();
Operand o = op.pop();
int result = calc(num1, o.op, num2);
num.push(result);
} else {
break;
}
}
op.push(new Operand(priority, ss[i]));
}
}
}
}
if(!n.equals("")) {
num.push(Integer.parseInt(n));
n = "";
}
while(!op.isEmpty()) {
int num2 = num.pop();
Operand o = op.pop();
int num1 = num.pop();
num.push(calc(num1, o.op, num2));
}
System.out.println(num.pop());
}
}
괄호를 고려하는 것이 조금 어려웠다.
'SW Academy' 카테고리의 다른 글
[CNU SW Academy] 17일차(22.12.23) (1) | 2022.12.23 |
---|---|
[CNU SW Academy] 코딩테스트 가이드 (0) | 2022.12.23 |
[CNU SW Academy] 14일차(22.12.20) (0) | 2022.12.23 |
[CNU SW Academy] 13일차(22.12.19) (0) | 2022.12.22 |
[CNU SW Academy] 12일차(22.12.16) (0) | 2022.12.18 |